Script Reference : Dialog
The Dialog class describes custom dialog box.
Now it is possible to create custom dialog with help of priPrinter script engine. Resource editor or dialog templates are not required. Script creates controls in dialog one by one.
Each control has name, id, value and control specific properties. Controls are positioned depending on text length/height.
In order to create new control, just call Dialog.Add method, provide control id and type. All additional properties should be passed in table. Value on any control can be defined during call to Add method or later. After executing dialog, value of any control can be retrieved.
Name | Type | Description |
---|---|---|
Title | string | String that specifies the title of dialog box. |
* | any type | If name matches ID of control, value of that control will be returned/changed. |
Name | Description |
---|---|
Dialog::Dialog() | Creates a Dialog object and initializes all data to defaults. |
Name | Return Type | Description |
---|---|---|
Insert(string id, string type, [Table]) | bool | Insert new control. Where Id is unique in control identifier. Currently following types are allowed: text, edit, checkbox, combobox, slider. Control properties are defined in table. Value is used to access control value. For list of control specific properties see table below. |
Button(string name, any type value) | bool | Insert new button. Name is defined by name parameter. Value will be returned by Execute function in case of pressing to this button. |
Execute() | any type | Execute dialog. Method returns value attached to custom button or null in case of Esc or system close button. |
Name | Type | Description |
---|---|---|
Common
value |
Control value. E.g. text in edit line or current slider position. | |
title | String | Name of the property. |
Text
font |
String, Font | In case of string allowed value is bold. In that case text font will standard bold font. Also it can be Font object. |
Edit
height |
Integer | Height of edit control in lines. 1 is default. |
suffix | String | Custom text which is placed after main control. It may be units of measurements for instance. |
type | String | Allowed values are: float, integer, string. |
min | float/integer | The min attribute specifies the minimum value of the range defined by the edit control. |
max | float/integer | The max attribute specifies the maximum value of the range defined by the edit control. |
step | float/integer | Step for up-down control. Used when type is set to float only. It's always 1 for integer controls. |
Checkbox
---- |
||
ComboBox editLine |
bool | By default, value can be selected from set of predefine items. When set to true, value can be edited in edit control (see help for CBS_DROPDOWN style). |
suffix | String | Custom text which is placed after main control. |
items | array of strings | |
Slider min |
float,integer | The min attribute specifies the minimum value of the range defined by the slider control. |
max | float,integer | The max attribute specifies the maximum value of the range defined by the slider control. |
suffix | String | Custom text which is placed after main control. You can include %1 in the text. %1 will be replaced with current value. |
Sample 1:
local dlg=Dialog (); dlg.title="Test Dialog"; local H0=Font("Segoe UI Light"); H0.Height=32; dlg.Insert("id_h0","text",{title="New Watermark",font=H0}); dlg.Insert("id_h1","text",{title="Type new text.\nText Description"}); dlg.Insert("id_value","edit",{title="Text:",height=10}); dlg.Insert("id_update","checkbox",{title="Update Existing",value=true}); dlg.id_value="New Text"; dlg.Button("OK",1); dlg.Button("Cancel",0); local a=dlg.Execute(); if(a) { print(dlg.id_value+" update:"+dlg.id_update+"\n"); }
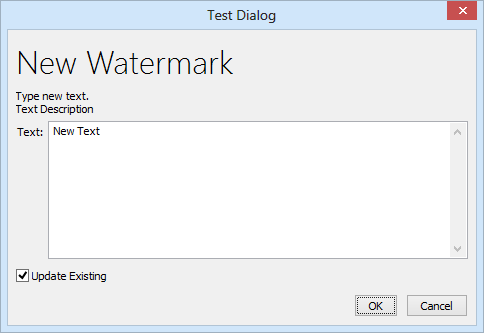
Sample 2:
local dlg=Dialog (); dlg.title="Configure Trays"; local H0=Font("Segoe UI Light"); H0.Height=24; local t=layout.GetTrays(); local a3=CfgGetValue("autotray/a3"); local a4=CfgGetValue("autotray/a4"); if(!a3.len())a3=layout.tray; if(!a4.len())a4=layout.tray; dlg.Insert("id_h0","text",{title="A3 Format",font=H0}); dlg.Insert("tray_a3","combobox",{title="Select Tray:",items=t,value=a3}); dlg.Insert("id_h1","text",{title="A4 Format",font=H0}); dlg.Insert("tray_a4","combobox",{title="Select Tray:",items=t,value=a4}); dlg.Insert("id_h1","text",{title="A3 Sheets will be printed on the first tray, A4 Sheets will go to second one."}); dlg.Button("OK",1); dlg.Button("Cancel",0); local a=dlg.Execute(); if(a==1) { CfgSetValue("autotray/a3",t[dlg.tray_a3]); CfgSetValue("autotray/a4",t[dlg.tray_a4]); }
ComboBox:
dlg.Insert("id_type","combobox", {title="Type:",items=["item1","item2","item3","item4","item5"],index=2,suffix="MM"});
Slider:
dlg.Insert("id_slider","slider",{title="Amount of space",value=0,suffix="KB",min=-1,max=2,suffix="%1 kb"});
Integer Edit line with up/down buttons:
dlg.Insert("id_numberI","edit",{title="Number I:",value=10,min=1,max=200,type="integer"});
Float edit line with up/down buttons:
dlg.Insert("id_numberF","edit",{title="Number F:",value=10,min=1,max=200,step=0.1,type="float"});